This is a small project
in android. In this project we simply play the sound when user press the
button. You can use this example in your android project.
Activity_main.xml
xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.programmingwap.playsound.MainActivity">
<TextView
android:id="@+id/txtView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello World!" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Play"
android:id="@+id/playBtn"
android:layout_centerVertical="true"
android:layout_centerHorizontal="true" />
</RelativeLayout>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.programmingwap.playsound.MainActivity">
<TextView
android:id="@+id/txtView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello World!" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Play"
android:id="@+id/playBtn"
android:layout_centerVertical="true"
android:layout_centerHorizontal="true" />
</RelativeLayout>
Main_activity.xml
package com.programmingwap.playsound; import android.media.MediaPlayer; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.TextView; public class MainActivity extends AppCompatActivity { Button playBtn; TextView textView; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); //intialize i.e Link with R playBtn = (Button) findViewById(R.id.playBtn); textView = (TextView)findViewById(R.id.txtView); playBtn.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { // Sound Function call here when user press the button playSound(); } }); } //function definition private void playSound(){ //crystall_ball is the name of the sound clip MediaPlayer player = MediaPlayer.create(this ,R.raw.crystal_ball); player.start(); player.setOnCompletionListener(new MediaPlayer.OnCompletionListener() { @Override public void onCompletion(MediaPlayer mp) { //Run those code when sound is off textView.setText("Sound Succesfully played"); } }); } }
Explanation:
· You modify the crystal_ball with your audio file.
Audio file pasted in res->raw->your_audio_file.mp3
MediaPlayer player = MediaPlayer.create(this ,R.raw.crystal_ball);
· setOnCompletionListener() is a method which absorb the completiton of the sound.
When sound played then all the code within this block run.
player.setOnCompletionListener(new MediaPlayer.OnCompletionListener() { @Override public void onCompletion(MediaPlayer mp) { //Run those code when sound is off textView.setText("Sound Succesfully played"); } });
Screenshot:

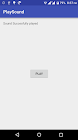
0 comments:
Post a Comment